Hi All,
I have created this blog for calculate BMI(Body mass Index) for both men and women. Its a rare combinations to get BMI for both men and women. we can give input in terms of Feet/Inches-Lbs or Cms/Kgs. lets start how to create BMI calculator using android program.
step 1: Create a new android project.
File->New->Project->Android->Android application project
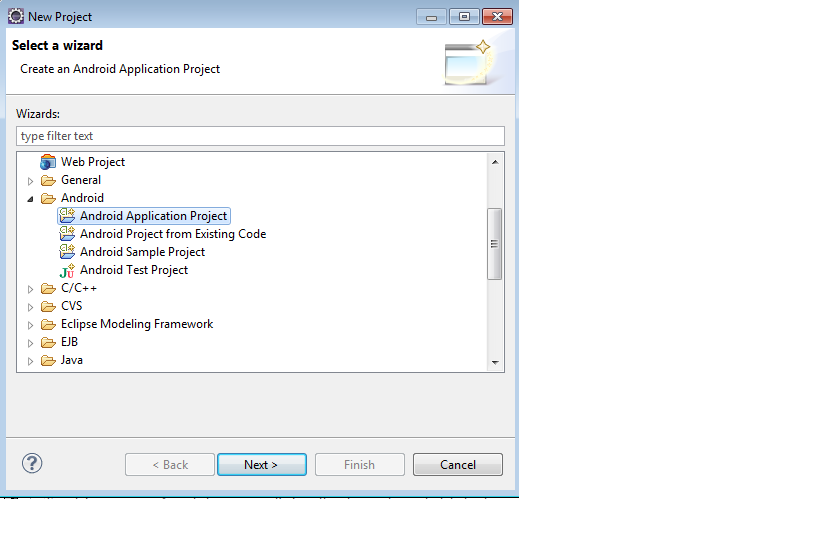
step 2: Create a new relative layout and add widgets(Edit text, text field,spinner,button)
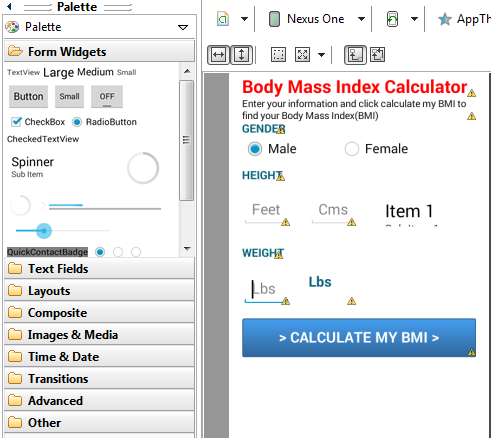
I have created this blog for calculate BMI(Body mass Index) for both men and women. Its a rare combinations to get BMI for both men and women. we can give input in terms of Feet/Inches-Lbs or Cms/Kgs. lets start how to create BMI calculator using android program.
step 1: Create a new android project.
File->New->Project->Android->Android application project
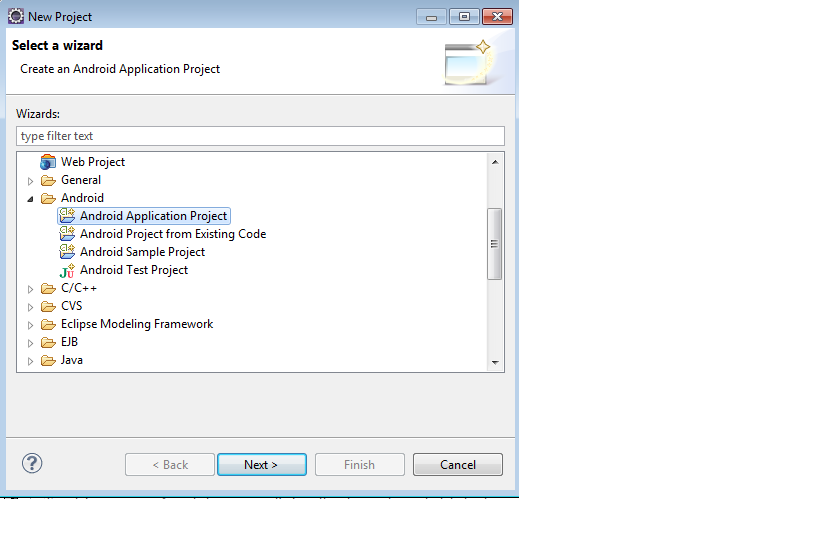
step 2: Create a new relative layout and add widgets(Edit text, text field,spinner,button)
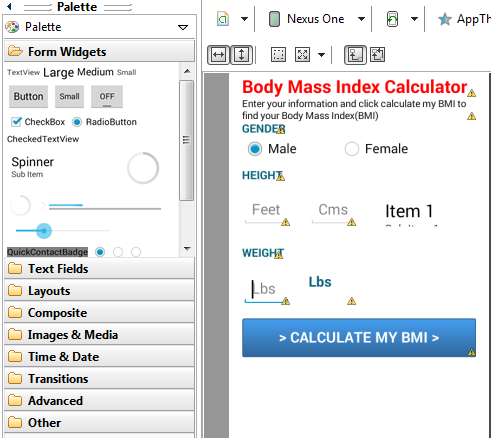
Else copy the below code for layout:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity" >
<TextView
android:id="@+id/textGender"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/textsubheading"
android:layout_below="@+id/textsubheading"
android:text="GENDER"
android:textColor="#055F7A"
android:textStyle="bold" />
<TextView
android:id="@+id/textHeading"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentRight="true"
android:layout_alignParentTop="true"
android:text="Body Mass Index Calculator"
android:textAppearance="?android:attr/textAppearanceLarge"
android:textColor="#ff0000"
android:textSize="22sp"
android:textStyle="bold" />
<TextView
android:id="@+id/textsubheading"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/radiosex"
android:layout_below="@+id/textHeading"
android:text="Enter your information and click calculate my BMI to find your Body Mass Index(BMI)"
android:textAppearance="?android:attr/textAppearanceMedium"
android:textSize="12sp" />
<RadioGroup
android:id="@+id/radiosex"
android:layout_width="300dp"
android:layout_height="40dp"
android:layout_alignParentLeft="true"
android:layout_below="@+id/textGender"
android:orientation="horizontal" >
<RadioButton
android:id="@+id/radiomale"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="0.05"
android:checked="true"
android:text="@string/radio_male" />
<RadioButton
android:id="@+id/radiofemale"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="0.08"
android:text="@string/radio_female" />
</RadioGroup>
<TextView
android:id="@+id/textheight"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/radiosex"
android:layout_alignRight="@+id/textGender"
android:layout_below="@+id/radiosex"
android:text="HEIGHT"
android:textColor="#055F7A"
android:textStyle="bold" />
<EditText
android:id="@+id/feet"
android:layout_width="60dp"
android:layout_height="40dp"
android:layout_alignLeft="@+id/textheight"
android:layout_below="@+id/textheight"
android:layout_marginTop="14dp"
android:hint="Feet"
android:ems="10"
android:inputType="numberDecimal" />
<EditText
android:id="@+id/cms"
android:layout_width="60dp"
android:layout_height="40dp"
android:layout_alignBaseline="@+id/feet"
android:layout_alignBottom="@+id/feet"
android:layout_marginLeft="28dp"
android:layout_toRightOf="@+id/textheight"
android:ems="10"
android:hint="Cms"
android:inputType="numberDecimal" />
<Spinner
android:id="@+id/heightspin"
android:layout_width="120dp"
android:layout_height="50dp"
android:layout_alignBottom="@+id/cms"
android:layout_alignRight="@+id/radiosex"
android:layout_alignTop="@+id/cms"
android:entries="@array/height_arrays" />
<TextView
android:id="@+id/textweight"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/feet"
android:layout_marginTop="24dp"
android:text="WEIGHT"
android:textColor="#055F7A"
android:textStyle="bold" />
<EditText
android:id="@+id/lbs"
android:layout_width="60dp"
android:layout_height="40dp"
android:layout_alignParentLeft="true"
android:layout_alignRight="@+id/feet"
android:layout_below="@+id/textweight"
android:layout_marginTop="16dp"
android:ems="10"
android:hint="Lbs"
android:inputType="numberDecimal" >
<requestFocus />
</EditText>
<TextView
android:id="@+id/Lbstext"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignBottom="@+id/lbs"
android:layout_alignLeft="@+id/cms"
android:layout_alignRight="@+id/cms"
android:layout_alignTop="@+id/lbs"
android:text="Lbs"
android:textSize="17sp"
android:textColor="#055F7A"
android:textStyle="bold" />
<Button
android:id="@+id/claculate"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/lbs"
android:layout_alignRight="@+id/heightspin"
android:layout_below="@+id/lbs"
android:layout_marginTop="15dp"
android:background="@drawable/button"
android:text="> CALCULATE MY BMI >"
android:textColor="#ffffff"
android:textStyle="bold" />
<TextView
android:id="@+id/result"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/claculate"
android:layout_alignRight="@+id/claculate"
android:layout_below="@+id/claculate"
android:text=""
android:textAppearance="?android:attr/textAppearanceMedium"
android:textSize="15sp" />
</RelativeLayout>
Step 3: Your string.xml file should look like,
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string name="app_name">BMI</string>
<string name="action_settings">Settings</string>
<string name="radio_male">Male</string>
<string name="radio_female">Female</string>
<string name="hello_world">Hello world!</string>
<string-array name="height_arrays">
<item>Feet/Inches</item>
<item>Cms</item>
</string-array>
</resources>
Step 4: Copy the below code and paste it in your main activity,
package com.example.bmiinfinite;
import android.os.Bundle;
import android.app.ActionBar;
import android.app.Activity;
import android.graphics.Color;
import android.graphics.drawable.ColorDrawable;
import android.widget.RadioButton;
import android.widget.RadioGroup;
import android.widget.Spinner;
import android.widget.TextView;
import android.widget.Toast;
import android.widget.Button;
import android.widget.EditText;
import android.view.View;
import android.widget.AdapterView;
import android.widget.AdapterView.OnItemSelectedListener;
import android.widget.ArrayAdapter;
public class MainActivity extends Activity {
private Spinner heightspin;
private EditText feet;
private EditText cms;
private EditText lbs;
private TextView Lbstext;
private TextView result;
private RadioGroup rg;
private RadioButton radioSexButton;
public int hfeet;
public int hinc;
public int wlbs;
public int wkgs;
public int hcms;
public float bmifeet;
public float bmicms;
public String bmifeetStatus;
public String bmicmsstatus;
public int selectedId;
public String spintext;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ActionBar bar = getActionBar();
bar.setBackgroundDrawable(new ColorDrawable(Color.parseColor("#c0c0c0")));
heightspin = (Spinner) findViewById(R.id.heightspin);
feet = (EditText) findViewById(R.id.feet);
cms = (EditText) findViewById(R.id.cms);
lbs = (EditText) findViewById(R.id.lbs);
Lbstext = (TextView ) findViewById(R.id.Lbstext);
result = (TextView ) findViewById(R.id.result);
rg = (RadioGroup) findViewById(R.id.radiosex);
spintext= heightspin.getSelectedItem().toString();
ArrayAdapter<CharSequence> adapter = ArrayAdapter.createFromResource(this,
R.array.height_arrays, android.R.layout.simple_spinner_item);
adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
heightspin.setAdapter(adapter);
heightspin.setOnItemSelectedListener(new OnItemSelectedListener() {
@Override
public void onItemSelected(AdapterView<?> parent, View view, int position, long id) {
if(position ==0 )
{
feet.setVisibility(View.VISIBLE);
cms.setHint("Inches");
Lbstext.setText("Lbs");
lbs.setHint("Lbs");
}
else
{
feet.setVisibility(View.GONE);
cms.setHint("Cms");
Lbstext.setText("Kgs");
lbs.setHint("Kgs");
}
}
@Override
public void onNothingSelected(AdapterView<?> arg0) {
// TODO Auto-generated method stub
}
});
final Button button = (Button) findViewById(R.id.claculate);
button.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
if (v.getId() == R.id.claculate )
{
EditText feet = (EditText)findViewById(R.id.feet);
EditText inches = (EditText)findViewById(R.id.cms);
EditText lbs = (EditText)findViewById(R.id.lbs);
result = (TextView ) findViewById(R.id.result);
hfeet = Integer.parseInt(feet.getText().toString());
hinc = Integer.parseInt(inches.getText().toString());
wlbs = Integer.parseInt(lbs.getText().toString());
selectedId = rg.getCheckedRadioButtonId();
radioSexButton = (RadioButton) findViewById(selectedId);
if(spintext.equalsIgnoreCase("Feet/Inches") && selectedId == R.id.radiomale)
{
bmifeet = lbsbmi(hfeet, hinc ,wlbs);
bmifeetStatus = staBMI(bmifeet);
result.setText("PRESENT STATUS :"+ String.format( "%.2f", bmifeet ) + "-" + bmifeetStatus);
}
if(spintext.equalsIgnoreCase("Feet/Inches") && selectedId == R.id.radiofemale)
{
bmifeet = lbsbmi(hfeet, hinc ,wlbs);
bmicmsstatus = fstaBMI(bmifeet);
result.setText("PRESENT STATUS :"+ String.format( "%.2f", bmifeet ) + "-" + bmicmsstatus);
}
if(spintext.equalsIgnoreCase("Cms") && selectedId == R.id.radiomale)
{
bmicms = kgscbmi(hcms, wkgs);
bmifeetStatus = staBMI(bmicms);
result.setText("PRESENT STATUS :"+ String.format( "%.2f", bmicms ) + "-" + bmifeetStatus);
}
if(spintext.equalsIgnoreCase("Cms") && selectedId == R.id.radiofemale)
{
bmicms = kgscbmi(hinc, wlbs);
bmicmsstatus = fstaBMI(bmicms);
result.setText("PRESENT STATUS :"+ String.format( "%.2f", bmicms ) + "-" + bmicmsstatus);
}
else
Toast.makeText(getApplicationContext(), "Please Put the values",
Toast.LENGTH_LONG).show();
}
}
});
}
private float lbsbmi(int feet, int inches, int lbs )
{
int inc= feet + (inches/12);
return (float) (lbs * 4.88 / (inc * inc));
}
private float kgscbmi (float cms, float kgs)
{
float hgt = cms/100 ;
return (float) ( kgs / (hgt * hgt));
}
private String staBMI(float bmiValue)
{
if (bmiValue < 18.5) {
return "Underweight";
}
else if (bmiValue < 25) {
return "Normal";
} else if (bmiValue < 30) {
return "Overweight";
} else {
return "Obese";
}
}
private String fstaBMI(float bmiValue)
{
if (bmiValue < 16.5) {
return "Underweight";
}
else if (bmiValue < 22) {
return "Normal";
} else if (bmiValue < 27) {
return "Overweight";
} else {
return "Obese";
}
}
}
step 5: create a new emulator by sdk manager,
Step 6: Run your project on BMI emulator.
Here we are giving background color ar run-time using the below code, user may choose any color any paste it.
ActionBar bar = getActionBar();
bar.setBackgroundDrawable(new ColorDrawable(Color.parseColor("#c0c0c0")));
Should you have any questions
on above pl feel free to contact undersigned
Happy reading!
Thanks,
Divakar U
GOOD
ReplyDeleteI can set up my new idea from this post. It gives in depth information. Thanks for this valuable information for all,.. cheat engine for mac,
ReplyDeleteI was just scrutinizing through the web hunting down a few information and kept running over your blog. I am motivated by the information that you have on this blog. It exhibits how well you appreciate this subject. Bookmarked this page, will return for extra.bmi chart for kids
ReplyDeleteThe 8 BEST Casino Bonus Codes in Kenya 2021 | JMTH
ReplyDelete› bonus-codes › bonus-codes The 8 여수 출장안마 BEST Casino Bonus Codes in Kenya 2021 · 1. Joka Casino · 2. Nubet Casino 순천 출장마사지 · 3. Slots 성남 출장샵 for Free · 4. 성남 출장마사지 BoBet Casino · 5. 부천 출장샵